Sessions
What is an agent session?
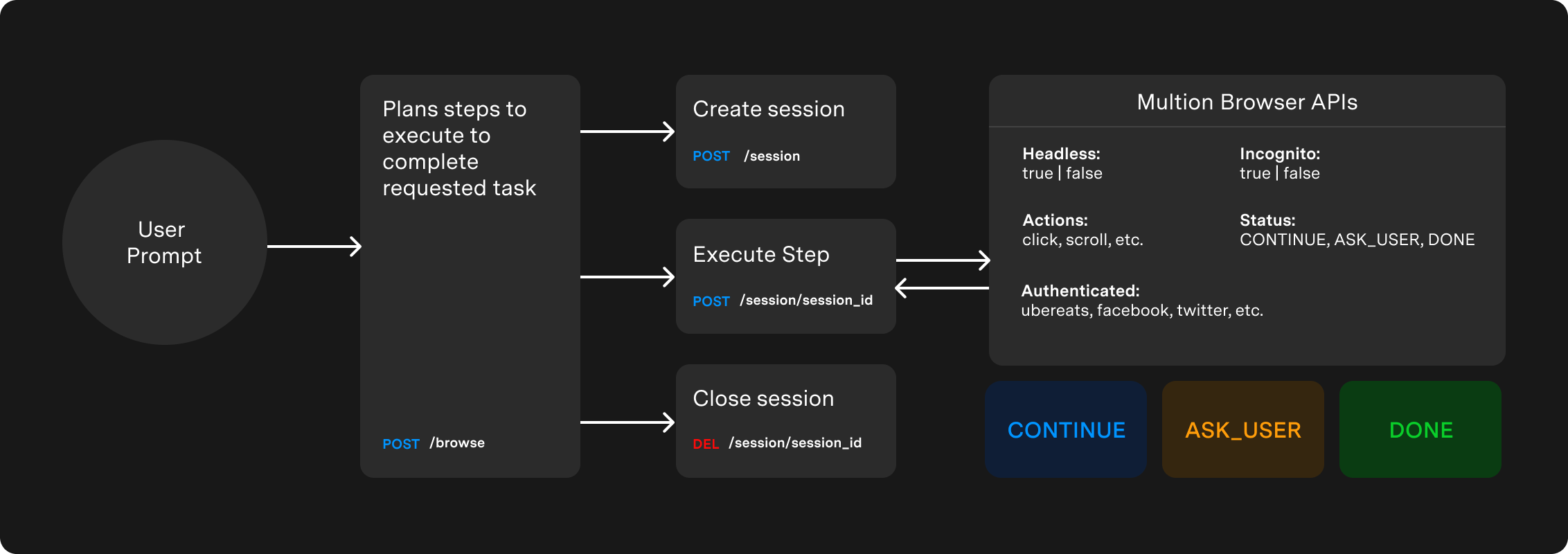
An agent session is a stateful instance of interaction with our agent for a specific user query or workflow. Each agent session is fully isolated and can be used to perform multiple sequential steps. When a session is created with a command, the agent generates a plan to complete the command.
With browse, the agent will step automatically until the action is completed or the agent requires additional input. With step session, you can supply additional information to refine the plan and help the agent at each step.
- Session lifecycle: An agent session begins when you initiate it with an input URL and an instruction prompt and ends when it has been closed or the session has expired. A session survives for 10 minutes if left inactive.
- Local mode: By default, sessions are hosted remotely in the cloud using our virtual headless browser. In local mode, our server-hosted agent interacts with your installed Chrome browser extension. Learn more here.
- Security: In remote mode, each agent session is fully isolated, protecting the integrity of your data and interactions.
Browse automatically
Browse allows you to automatically create an agent session with a URL and a command. It will step through the session until the action is completed or the agent requires additional input.
Create a session manually
Create session allows you to manually create an agent session with a URL. You can continue the session using the session_id
returned in the response.
Local mode
Use the local
flag to run the agent locally on your browser. Make sure the browser extension is installed and API Enabled is checked.
Speed modes
Use the mode
param to specify the speed model of the agent. This setting determines which LLM model is used under the hood, affecting the balance between processing speed and task complexity:
standard
: Optimized for complex tasks that require more detailed analysis or multi-step reasoning.fast
: Suitable for general tasks and quicker responses, ideal for simpler queries or when speed is a priority.
Choose the mode that best fits your specific use case and performance requirements.
Use proxy
Use the use_proxy
flag to enable proxy for the session to bypass IP blocks and bot protections. When enabled, the agent will be slightly slower to respond.
Step through a session
Step session allows you to step through an agent session with a command. It will return a response with the status of the agent. Status can be one of CONTINUE
, ASK_USER
, and DONE
. If the status is ASK_USER
, you can provide additional information to the agent in the cmd
field.
You can keep stepping through the session until the status is ASK_USER
or DONE
.
Get session screenshot
Use the include_screenshot
flag to include a screenshot URL of the session in the response.
Close a session manually
To close a session before it expires, use close session.